Description | Sample |
---|
Prevent Refreshing Table Control | function init(){ var oTable = getControl("vendorListT"); oTable.setNoAutoLoad(true); |
Trigger action on select record in a table | oTable.attachSelect(function(evt){ tableRowSelected(evt); });
function tableRowSelected(evt){ console.log("table Row Selected"); var recordChosen = evt.getParameter("listItem").getBindingContext().getObject(); console.log(recordChosen.vendorName); if(recordChosen.vendorName!=="(Vendor not in the list. Request new vendor)"){ //setField("vendorDetailsVisibile","true"); //Check if possible to extend to cc var companyCode = getField("DFSICompanyCode"); initModel("searchhelps","/sap/opu/odata/sap/ZFAB_TS_SERVICESN");
var oModel = getModel("searchhelps"); var filters = []; var oFilter = new sap.ui.model.Filter("Id", sap.ui.model.FilterOperator.EQ, recordChosen.vendorNo); filters.push(oFilter); var oFilterCC = new sap.ui.model.Filter("CC", sap.ui.model.FilterOperator.EQ, companyCode); filters.push(oFilterCC);
var oPath = "/VendorCCCheckList"; oModel.read(oPath, { success: function(data) { for(var i in data.results){ var oResult = data.results[i]; console.log(oResult.Result); jQuery.sap.require("sap.m.MessageBox"); var strHTML = "Vendor: " + oResult.Id + " - " + recordChosen.vendorName + "\n\n" + "Trading As: " + oResult.Name2 + "\n\n" + "Trading As: " + oResult.Name3 + "\n\n" + "Street: " + oResult.Street + "\n\n" + "City: " + oResult.City + "\n\n" + "State: " + oResult.State + "\n\n" + "Country: " + oResult.Country + "\n\n" + "PO Box: " + oResult.PO + "\n\n" + "PO Box City: " + oResult.POCity + "\n\n" + "PO Box State: " + oResult.POState + "\n\n" + "PO Box Country: " + oResult.POCountry + "\n\n" + "Blocked: " + oResult.Blocked + "\n\n" + "Purchasing Blocked: " + oResult.PBlocked + "\n\n" + "Deleted: " + oResult.Deleted; sap.m.MessageBox.show(strHTML , { title: "Vendor Details", onClose: null }); setField("EVendorNo",recordChosen.vendorNo); setField("vname1",recordChosen.vendorName); setField("name2",oResult.Name2); setField("name3",oResult.Name3); setField("searchTerm1",oResult.STerm1); setField("industry",oResult.Industry); setField("centralBlockIndicator",oResult.Blocked); setField("purchasingBlockIndicator",oResult.PBlocked); if(oResult.Result=="CC Exists"){ setField("unableExtendVisible", "true"); setField("extendVendorVisibile", "false"); setField("newVendorVisible", "false"); setField("newVendFieldVisible", "false"); setField("formShow", "false"); setField("extendVendFieldVisible", "false"); setField("newVendFieldEnabled", "false"); setField("CommentsVisible", "false"); }else{ setField("unableExtendVisible", "false"); setField("extendVendorVisibile", "true"); setField("newVendorVisible", "false"); setField("newVendFieldVisible", "false"); setField("formShow", "false"); setField("extendVendFieldVisible", "false"); setField("newVendFieldEnabled", "false"); setField("CommentsVisible", "false"); } } }, filters:filters }); }else{ setField("EVendorNo",""); setField("vname1",""); setField("name2",""); setField("name3",""); setField("searchTerm1",""); setField("vendorDetailsVisibile","false"); setField("newVendorVisible","true"); setField("unableExtendVisible","false"); setField("extendVendorVisibile","false"); setField("newVendFieldVisible", "false"); setField("formShow", "false"); setField("extendVendFieldVisible", "false"); setField("newVendFieldEnabled", "false"); } }; |
Set Max Length in an Input field | getControl("VendorNameId").setProperty("maxLength",40); |
Call oData from script | initModel("searchhelps","/sap/opu/odata/sap/ZFAB_TS_SERVICESN"); var oModel = getModel("searchhelps"); var oPath = "/BankList"; oModel.read(oPath, { success: function(data) { _bsb = data.results; } }); |
Bind Visibility Property | getControl("ApproverErrorId").getParent().bindProperty("visible","ApproverError"); |
Validate Email Input Field | function validateEmail(evt){ var testEmail = getField("emailVendorAddress"); var replaced = testEmail.split(' ').join(''); testEmail = replaced; if (/^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/g.test(testEmail)){ //if (^\w+([.-]\w+)*@\w+([.-]\w+)*(\.\w{2,4})+$/g.test(testEmail)){ setField("emailVendorAddress", testEmail); return true; } else { jQuery.sap.require("sap.m.MessageBox"); sap.m.MessageBox.show( "Invalid Purchase Order email address, example format-jsmith@example.com.au", "ERROR", "Validation Error", "OK", function(evt){ getControl("emailVendorAddressId").setValueState("Error"); } ); return false; } }; |
Calling *SAP Search Help using JavaScript | 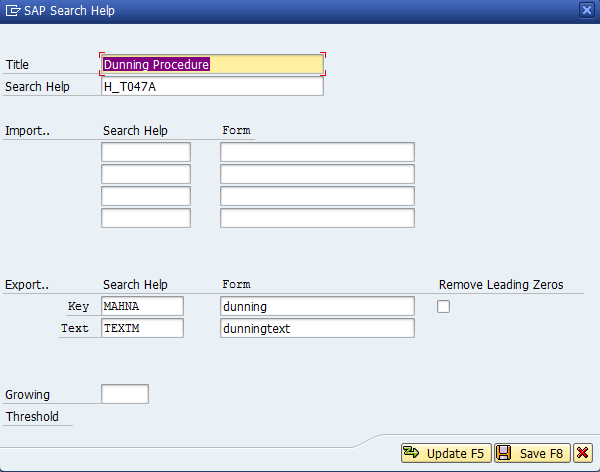 initModel("searchhelps","/sap/opu/odata/iqx/SERVICES_SRV"); var oModel = getModel("main"); var filters = []; var oFilter = new sap.ui.model.Filter("Config", sap.ui.model.FilterOperator.EQ, "H_T047A::MAHNA,TEXTM:"); filters.push(oFilter); oFilter = new sap.ui.model.Filter("Name", sap.ui.model.FilterOperator.EQ, testText); filters.push(oFilter); var oPath = "/GenSearchHelp"; oModel.read(oPath, { success: function(data) { for(var i in data.results){ var oResult = data.results[i]; setField("dunningtext",oResult.Name); found = "X"; } }, filters:filters }); |
Scroll to an element in a page | getControl("ReviewAndSubmit").scrollToElement(getControl("1Employeeid")); "ReviewAndSubmit" is the page ID and "1Employeeid" is the element ID within the page |
Highlight fields in the table | //Add style var oTableData = getControl("dataTable"); var oBinding = oTable.getBinding("items"); var length = oBinding.iLength; for(var i in length){ var oRow = oTableData.getItems()[i]; controlPosition = 1; //Position of the field in the column oControl = oRow.getCells()[controlPosition]; oControl.addStyleClass("myErrorState"); }
//Remove Style controlPosition = 1; //Position of the field in the column oControl = oRow.getCells()[controlPosition]; oControl.removeStyleClass("myErrorState");
|
Enable wrapping of label | Add in CSS: .sapMLabel { white-space: pre-wrap; } |
ABN Validation (Australia) | var d1,d2,d3,d4,d5,d6,d7,d8,d9,d10, d11, tot, rem, ok; var str = '51 824 753 556'; var replaced = str.split(' ').join(''); str = replaced; d1 = str.substr(0,1); parseInt(d1); if ( d1 > 0 ) { d1 = d1 - 1; } d1 = d1 * 10; d2 = str.substr(1,1) * 1; d3 = str.substr(2,1) * 3; d4 = str.substr(3,1) * 5; d5 = str.substr(4,1) * 7; d6 = str.substr(5,1) * 9; d7 = str.substr(6,1) * 11; d8 = str.substr(7,1) * 13; d9 = str.substr(8,1) * 15; d10 = str.substr(9,1) * 17; d11 = str.substr(10,1) * 19; tot = d1 + d2 + d3 + d4 + d5 + d6 + d7 + d8 + d9 + d10 + d11; rem = tot % 89; if ( rem === 0 ) { ok = 'true'; }else { ok = 'false'; } var d1,d2,d3,d4,d5,d6,d7,d8,d9,d10, d11, tot, rem, ok; var str = '51 824 753 556'; var replaced = str.split(' ').join(''); str = replaced; d1 = str.substr(0,1); parseInt(d1); if ( d1 > 0 ) { d1 = d1 - 1; } d1 = d1 * 10; d2 = str.substr(1,1) * 1; d3 = str.substr(2,1) * 3; d4 = str.substr(3,1) * 5; d5 = str.substr(4,1) * 7; d6 = str.substr(5,1) * 9; d7 = str.substr(6,1) * 11; d8 = str.substr(7,1) * 13; d9 = str.substr(8,1) * 15; d10 = str.substr(9,1) * 17; d11 = str.substr(10,1) * 19; tot = d1 + d2 + d3 + d4 + d5 + d6 + d7 + d8 + d9 + d10 + d11; rem = tot % 89; if ( rem === 0 ) { ok = 'true'; }else { ok = 'false'; } |
Add custom headers in page | function onLoad() { debugger; getControl("Page1").addStyleClass("pagebackgroundimage"); var oPage = getControl("Page2"); oPage.setCustomHeader(new sap.m.Bar({ contentLeft: [new sap.m.Button({ id: "_logOffButton", type: "Default", press: "onLogoutButtonPress", visible: false, tooltip: "Log Out", icon: "sap-icon://log" }), new sap.m.Button({ id: "_navButton", type: "Back", press: "backPressed", visible: true }) ], contentMiddle: [new sap.m.Label({ text: "Test", id: "_page2_title" })], contentRight: [new sap.m.Image({ src: "/sap/bc/bsp/sap/ZIQX_DEMO_THEME/iqxlogo-300x179.png", height: "40px" }), new sap.m.Label({ text: "Version 1.0", id: "Versionid" }) ] })); } |
Reading parameters from the URL | Sample URL
http://fiori.iqxbusiness.com/sap/bc/ui5_ui5/iqx/fab2/index_latest.html?sap-ui-appcache=false&interactive2=true&showlogoff=true&UserReference=10#Form/CN_CAPEX var UserReference = jQuery.sap.getUriParameters().get("UserReference");
|
Hide Back button on Review and Submit page | if (getField("CurrentStatus" == STATUS) { getControl("_view1–_navButton").setVisible(false); } |
Dynamically load 3rd party external lib from external | This helps loading external 3rd JS library when it is not available in FAB Code Block |
---|
language | js |
---|
linenumbers | true |
---|
| try {
var l_today = Date.today();
} catch (err) {
new Promise(function (fnResolve, fnReject) {
jQuery.sap.includeScript(
"https://cdnjs.cloudflare.com/ajax/libs/datejs/1.0/date.min.js",
"DateJSLib", fnResolve, fnReject);
}).then(function () {
console.log("lib loaded!!!")
});
} |
|
Get all the model data fields | Call the inbuilt function fabGetData() to fetch all the model data fields. var getData = fabGetData() |
Ternary Operator | Accepts 3 operands and acts as a shortcut to IF statements https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Conditional_Operator |
Get the current page | var currentPage = _fab.byId("_app").getCurrentPage().getId() You can compare it with getControl("YourPageName").getId() to check if its the same page you are doing validations on. |
Change the code while debugging to test | Add a line of code in the first script you use with the syntax //# sourceURL=some_name.js Eg: //# sourceURL=journal_upload.js This would open the journal_upload.js in runtime instead of the temporary application and enables the developer to change code on the fly to check while debugging. NOTE: This line of code has to be deleted before it is moved to production, as it gives a developer the ability to change code in the run-time. |